Blind Booleans vs. Context: Rethinking Boolean Returns
· Programming · Alejandro Cantero Jódar
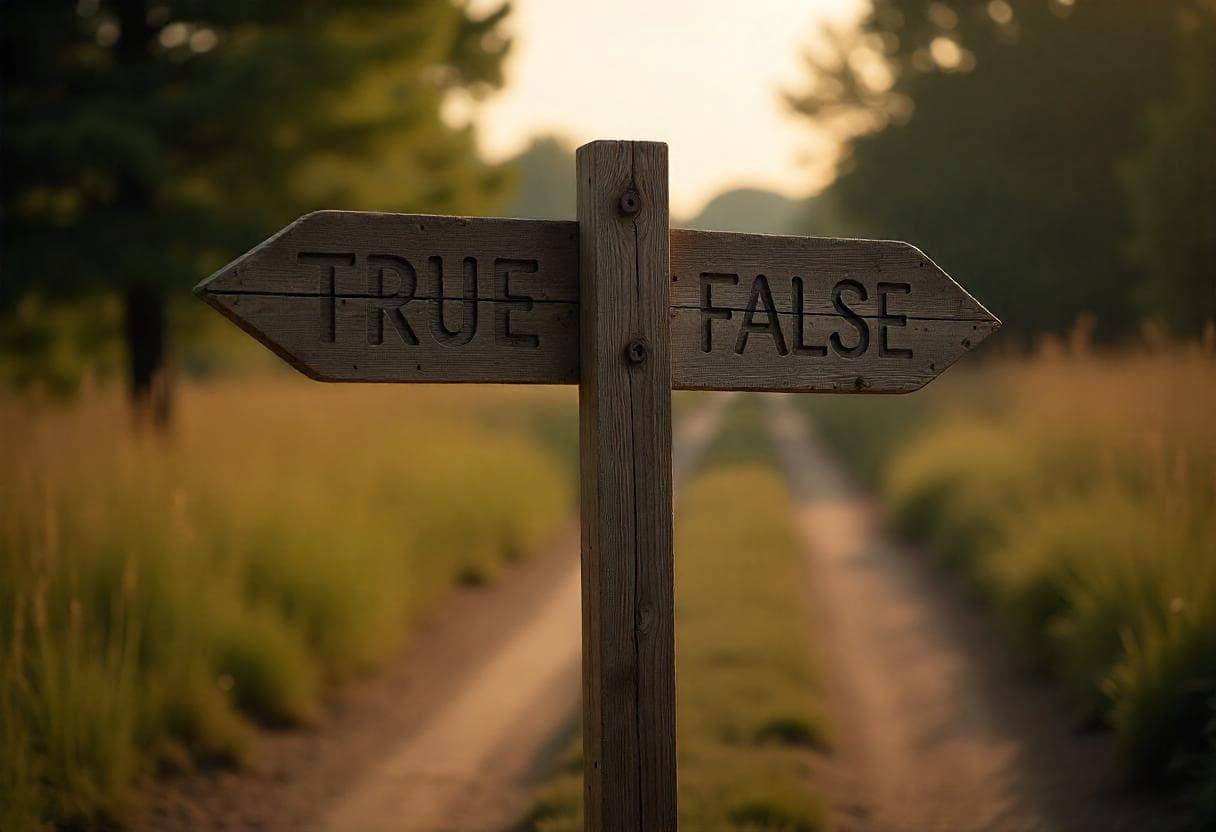
In the realm of programming, the use of boolean values—true and false—has been a longstanding practice. However, a growing discourse challenges the conventional use of booleans, particularly focusing on the concept of 'blind booleans' and advocating for returning objects that provide richer context. This article delves into this debate, exploring the arguments against blind booleans and presenting the case for more informative return values.
Understanding 'Blind Booleans'
A 'blind boolean' refers to a boolean value returned by a function without any accompanying context, making it ambiguous and potentially misleading. For instance, consider a function in JavaScript:
function isAdult(age) {
return age >= 18;
}
This function returns true
or false
based solely on the input age. While the function name provides some context, the returned boolean lacks explicit information about what true
or false
signifies. This ambiguity can lead to confusion, especially when the return value is used elsewhere in the code without clear documentation.
The term 'blind boolean' encapsulates this lack of context, highlighting the potential pitfalls of returning raw boolean values without additional information.
The Case Against Blind Booleans
Critics of blind booleans argue that they can lead to code that is harder to read, maintain, and debug. The primary concerns include:
Lack of Clarity: Without context, a boolean return value doesn't convey meaningful information. Developers must rely on function names or external documentation to understand the significance of the return value.
Increased Cognitive Load: When reading code, encountering a boolean return value without context forces developers to trace back to the function definition to grasp its meaning, thereby increasing cognitive load.
Potential for Misuse: Blind booleans can be easily misinterpreted or misused, leading to bugs and unintended behavior in the application.
As noted in an article on 30 Seconds of Code, boolean arguments in functions can be the source of a lot of wasted time and the cause for low code readability if used incorrectly. (30secondsofcode.org)
Advocating for Contextual Return Values
To address the shortcomings of blind booleans, many developers advocate for returning objects or enums that provide richer context. This approach enhances code readability and maintainability by making the return values self-explanatory.
Returning Objects with Context
Instead of returning a raw boolean, a function can return an object that includes the boolean value along with additional context. For example:
function getAdultStatus(age) {
return {
isAdult: age >= 18,
age: age,
message: age >= 18 ? 'User is an adult.' : 'User is not an adult.'
};
}
This approach provides a comprehensive understanding of the result, reducing ambiguity and enhancing code clarity.
Utilizing Enums for Clarity
In languages that support enums, replacing booleans with enums can make the code more expressive. For instance, in Swift:
enum AgeStatus {
case adult
case minor
}
func getAgeStatus(age: Int) -> AgeStatus {
return age >= 18 ? .adult : .minor;
}
This method eliminates the ambiguity associated with booleans, as the return value clearly indicates the age status.
Addressing Counterarguments
While the move away from blind booleans has its proponents, it's essential to consider counterarguments:
Simplicity vs. Verbosity: Some argue that introducing objects or enums for simple true/false conditions adds unnecessary complexity. In cases where the boolean's meaning is evident and well-documented, returning a boolean might suffice.
Performance Considerations: Returning objects instead of booleans can have performance implications, especially in performance-critical applications. However, in most high-level programming scenarios, the impact is negligible.
It's crucial to strike a balance between clarity and simplicity, assessing each case individually to determine the most appropriate approach.
Practical Examples and Case Studies
Consider a function that checks if a user has administrative privileges:
function hasAdminAccess(user) {
return user.role === 'admin';
}
This function returns a boolean without context. A more informative approach would be:
function getAccessLevel(user) {
return {
isAdmin: user.role === 'admin',
role: user.role,
permissions: getPermissionsForRole(user.role)
};
}
This method provides a comprehensive view of the user's access level, reducing ambiguity and potential errors.
In a case study discussed by Matt Diephouse, replacing booleans with enums improved code clarity and correctness, demonstrating the practical benefits of this approach. (matt.diephouse.com)
Quick Takeaways
Blind booleans are boolean values returned without context, leading to ambiguity and potential misuse.
Returning objects or enums with context enhances code readability and maintainability.
While adding context is beneficial, it's essential to balance clarity with simplicity to avoid unnecessary complexity.
Assess each function individually to determine the most appropriate return type based on its specific requirements.
Conclusion
The debate over the use of booleans in programming underscores the importance of clarity and context in code. While booleans are fundamental, their blind use can lead to ambiguous and error-prone code. By returning objects or enums that provide richer context, developers can create more readable, maintainable, and robust applications. As with many programming practices, the key lies in thoughtful consideration and balancing simplicity with clarity.
FAQs
Q: What is a 'blind boolean' in programming?
A 'blind boolean' is a boolean value returned by a function without any accompanying context, making it ambiguous and potentially misleading.
Q: Why are blind booleans considered problematic?
Blind booleans can lead to code that is harder to read, maintain, and debug due to their lack of clarity and context.
Q: How can returning objects instead of booleans improve code quality?
Returning objects provides additional context, making the code more informative and reducing ambiguity, which enhances readability and maintainability.
Q: Are there situations where returning a boolean is appropriate?
Yes, in cases where the boolean's meaning is evident and well-documented, returning a boolean might suffice. It's essential to assess each case individually.
Q: Does replacing booleans with objects or enums impact performance?
While there might be a slight performance impact, in most high-level programming scenarios, the difference is negligible compared to the benefits in code clarity and maintainability.
So, do you prefer returning booleans or objects with context in your functions?